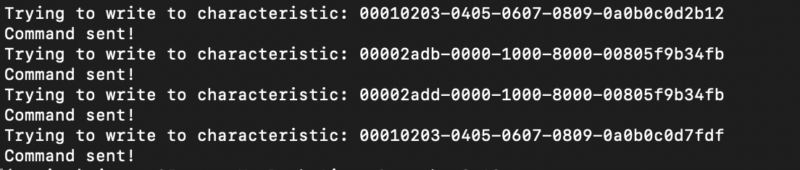
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
import asyncio from bleak import BleakClient # Device address DEVICE_ADDRESS = "adfafds-adfadfs-adfadsf-afafd-afdasfdsdf" # UUIDs for characteristics that might control the light CHARACTERISTIC_UUIDS = [ "00010203-0405-0607-0809-0a0b0c0d2b12", # Service 1 "00002adb-0000-1000-8000-00805f9b34fb", # Service 2 "00002add-0000-1000-8000-00805f9b34fb", # Service 3 "00010203-0405-0607-0809-0a0b0c0d7fdf" # Service 4 ] async def turn_on_light(client): # The command to turn on the light. The exact command may differ. # This is a placeholder; you'll need to know the correct byte sequence for your device. turn_on_command = bytearray([0x01]) for characteristic_uuid in CHARACTERISTIC_UUIDS: try: print(f"Trying to write to characteristic: {characteristic_uuid}") await client.write_gatt_char(characteristic_uuid, turn_on_command, response=False) print("Command sent!") except Exception as e: print(f"Failed to write to {characteristic_uuid}: {e}") async def main(): # Create a BleakClient instance with the device address async with BleakClient(DEVICE_ADDRESS) as client: print(f"Connected to {client.address}") # Try to turn on the light await turn_on_light(client) if __name__ == "__main__": asyncio.run(main()) |