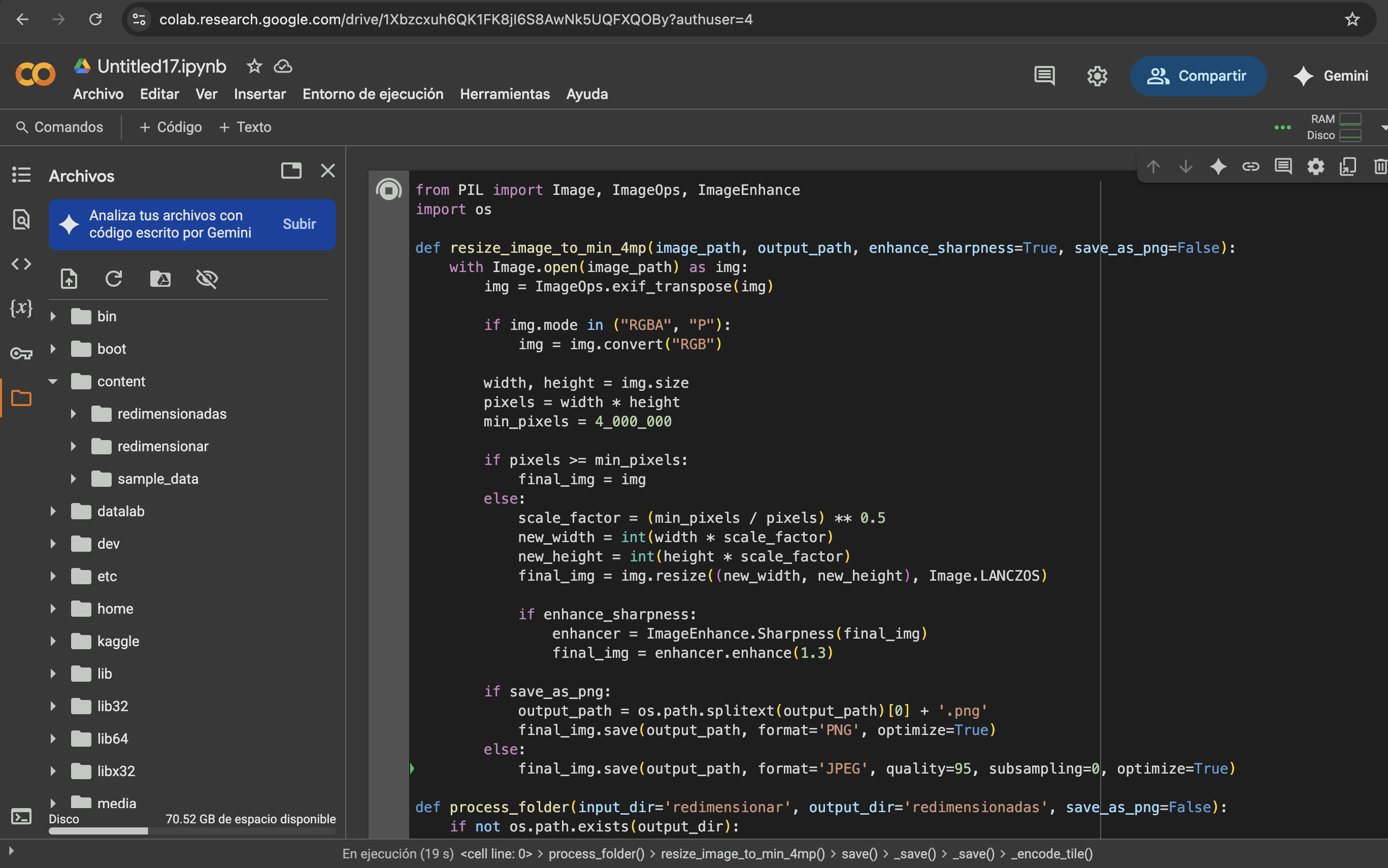
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
from PIL import Image, ImageOps, ImageEnhance import os def resize_image_to_min_4mp(image_path, output_path, enhance_sharpness=True, save_as_png=False): with Image.open(image_path) as img: img = ImageOps.exif_transpose(img) if img.mode in ("RGBA", "P"): img = img.convert("RGB") width, height = img.size pixels = width * height min_pixels = 4_000_000 if pixels >= min_pixels: final_img = img else: scale_factor = (min_pixels / pixels) ** 0.5 new_width = int(width * scale_factor) new_height = int(height * scale_factor) final_img = img.resize((new_width, new_height), Image.LANCZOS) if enhance_sharpness: enhancer = ImageEnhance.Sharpness(final_img) final_img = enhancer.enhance(1.3) if save_as_png: output_path = os.path.splitext(output_path)[0] + '.png' final_img.save(output_path, format='PNG', optimize=True) else: final_img.save(output_path, format='JPEG', quality=95, subsampling=0, optimize=True) def process_folder(input_dir='redimensionar', output_dir='redimensionadas', save_as_png=False): if not os.path.exists(output_dir): os.makedirs(output_dir) supported_extensions = ('.jpg', '.jpeg', '.png', '.bmp', '.tiff', '.webp') for filename in os.listdir(input_dir): if filename.lower().endswith(supported_extensions): input_path = os.path.join(input_dir, filename) output_path = os.path.join(output_dir, filename) print(f"🔄 Procesando: {filename}") try: resize_image_to_min_4mp(input_path, output_path, enhance_sharpness=True, save_as_png=save_as_png) print(f"✅ Guardado: {os.path.basename(output_path)}\n") except Exception as e: print(f"⚠️ Error con {filename}: {e}\n") # Ejecutar process_folder() |